由于项目需求,需要从传感器节点和GPS中读取数据,所以学习了如何用python读串口。
python提供了pySerial,Linux和windows下有相应的模块
linux版本: pyserial (http://pyserial.wiki.sourceforge.net/pySerial)
(在我的虚拟机中Ubuntu 12.04 LTS 版本中好像已经自带)
windows版本: pywin32 (http://sourceforge.net/projects/pywin32/)
下载直接安装
先看一个例子:
import serial
ser = serial.Serial('/dev/ttyTX0', 9600, timeout=1)
while True:
data = ser.read(100)
print repr(data)
1、读串口步骤:
实例化 --> 设置 (当然可以一步完成)
2、serial详解
2.1 serial类原型
ser = serial.Serial(
port=None, # number of device, numbering starts at
# zero. if everything fails, the user
# can specify a device string, note
# that this isn't portable anymore
# if no port is specified an unconfigured
# an closed serial port object is created
baudrate=9600, # baud rate
bytesize=EIGHTBITS, # number of databits
parity=PARITY_NONE, # enable parity checking
stopbits=STOPBITS_ONE, # number of stopbits
timeout=None, # set a timeout value, None for waiting forever
#zero, non-blocking mode, return immediately on read
xonxoff=0, # enable software flow control
rtscts=0, # enable RTS/CTS flow control
interCharTimeout=None # Inter-character timeout, None to disable
)
注;Port可以用数字表示。COM1为 0,COM2为1 .......
也可以实用绝对路径:ser.port = '/dev/ttySAc2'
timeout说明(None和 0不同):
timeout = None: 长时间等待
timeout = 0: 不阻塞形式 (读完之后就返回)
timeout = x: x秒后超时 (float allowed)
2.2 Serial方法:
open() # open port
close() # close port immediately
setBaudrate(baudrate) # change baud rate on an open port
inWaiting() # return the number of chars in the receive buffer
read(size=1) # read "size" characters
write(s) # write the string s to the port
flushInput() # flush input buffer, discarding all it's contents
flushOutput() # flush output buffer, abort output
sendBreak() # send break condition
setRTS(level=1) # set RTS line to specified logic level
setDTR(level=1) # set DTR line to specified logic level
getCTS() # return the state of the CTS line
getDSR() # return the state of the DSR line
getRI() # return the state of the RI line
getCD() # return the state of the CD line
2.3 Serial 实例属性
portstr # device name
BAUDRATES # list of valid baudrates
BYTESIZES # list of valid byte sizes
PARITIES # list of valid parities
STOPBITS # list of valid stop bit widths
以下属性可以改变,即使在端口打开时也可以重新赋值
port # port name/number as set by the user
baudrate # current baud rate setting
bytesize # byte size in bits
parity # parity setting
stopbits # stop bit with (1,2)
timeout # timeout setting
xonxoff # if Xon/Xoff flow control is enabled
rtscts # if hardware flow control is enabled
3.简单实例
import serial
ser = serial.Serial()
def hexShow(argv):
result = ''
hLen = len(argv)
for i in xrange(hLen):
hvol = ord(argv[i])
hhex = '%02x'%hvol
result += hhex+' '
print 'hexShow:',result
ser.baudrate = 57600
ser.port = '/dev/ttySAC2'
ser.open()
readstr = ser.read(20)
print ser.portstr
hexShow(readstr)
执行结果如图:
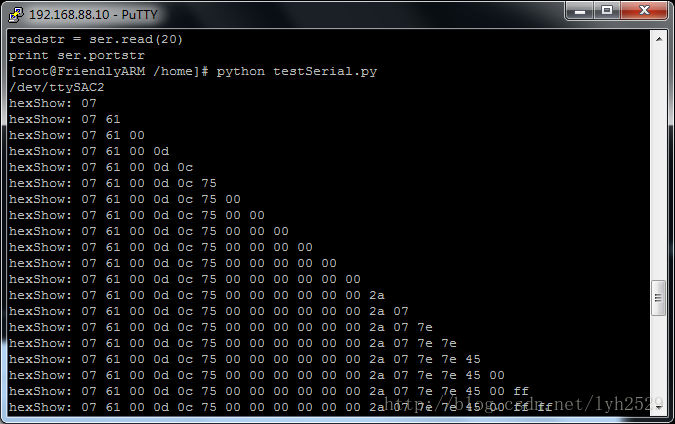